JavaScript fundamentals
HTML, CSS and JS
As I've mentioned in my last blog post, we need HTML to structure information, CSS - for making it look pretty and, finally, we need JavaScript to make a page alive - let the page visitors press buttons, play animations, show alerts and warnings, renew a content without leaving the page, process data from forms and many other things.
We can easily find an analogy in the real life. Let’s have a look look at the building. The structure of the building is HTML, wallpapers, wooden floors, paint and other decorations - CSS, utilities (water, electricity, landline etc) are JavaScript.
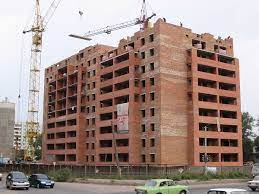
HTML
DOM - document object model
HTML and CSS are interpreted by browsers. What exactly does it mean? When you enter the page address the browser sends a request to a server (think about the server as a place on the internet where pages are stored). When the browser gets HTML text, it builds a structure, which looks like an upside-down tree. This tree calls DOM - Document Object Model.
Have a look at the example from the last blog post:
<html>
<head>
<link href="my-styles.css">
</head>
<body>
<h1 class="my-heading">I am a heading!</h1>
<p class="my-paragraph"> I am a paragraph. I am longer
than the heading and I contain a lot of very valuble and
useful information.</p>
<p class="my-paragraph">I am a paragraph too. I have the
same style as the previos one, but my personality
is different.</p>
</body>
</html>
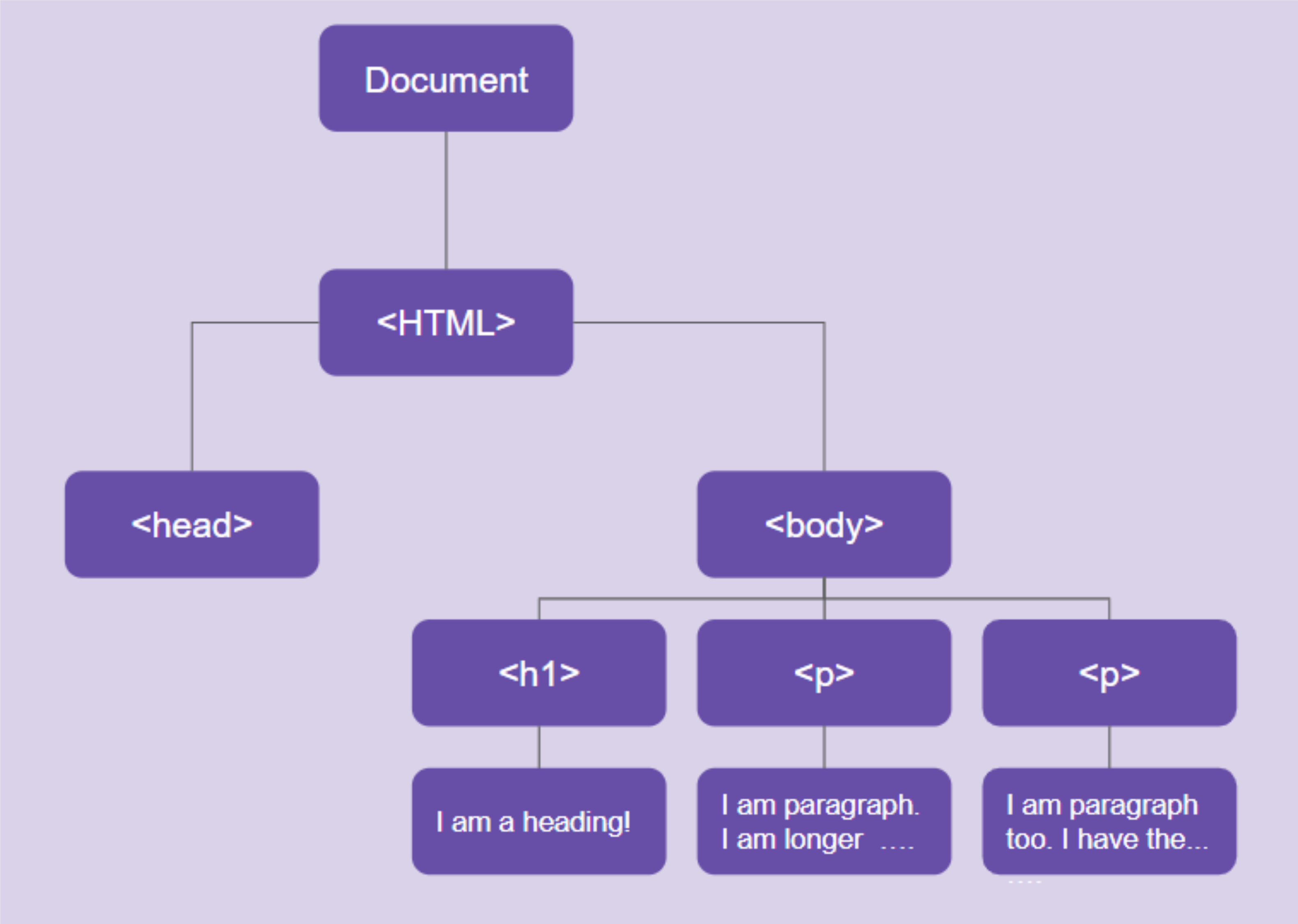
DOM
If you turn it upside down you will understand why they call it a tree:
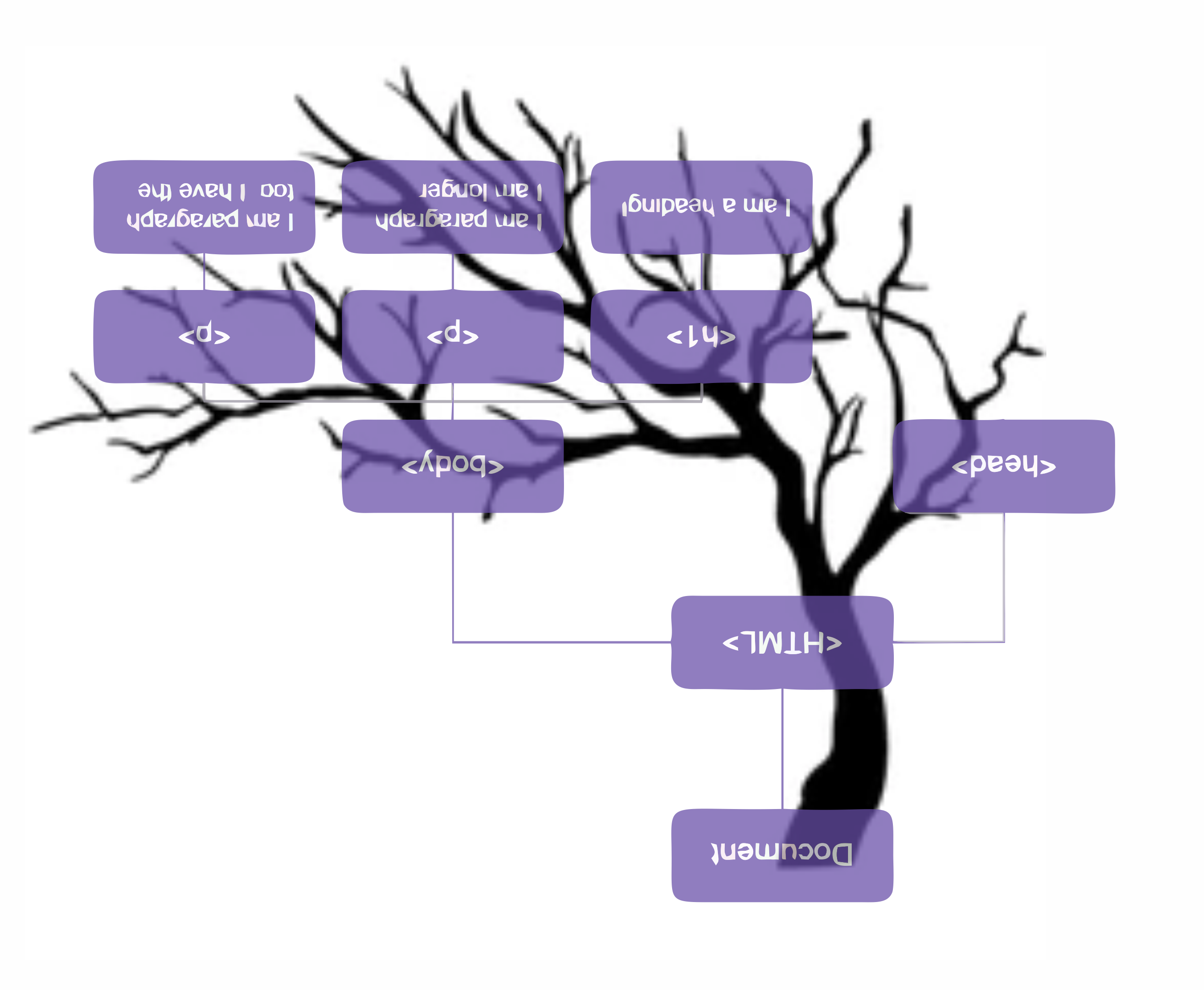
After DOM has been built the browser reads CSS styles and build an another tree - CSSOM. Those two trees combined make a final Render Tree which browser paints on the screen (more information about the render tree is here)..
Note: some elements can have “display” property set to “none”; which makes them invisible. Those elements will be in the DOM, but won’t be in the final Render Tree
Now the page is displayed and there is only one way to change something there - use JavaScript.
As you can see, JavaScript is an important part of web development. JavaScript is a programming language, unlike HTML which is a markup language. Programming languages are the whole world, there are different languages for different purposes: C++ is used for creating operation systems, games, browsers, applications etc, SQL - for working with databases, Swift - for creating apps for iOS and JavaScript is mostly used for web development.
Program languages have different syntaxes, but most of them use similar conceptions - variables, functions, arrays, loops etc. We will use our building analogy to dive deeper and understand some of the fundamentals of JavaScript.
Fundamentals of JS
Imagine we have a building (HTML) with all pretty walls and floors (CSS applied). We have some furniture in the building already (out texts and images) and a truck with more furniture has just arrived. We are waiting for a delivery from IKEA, but on the truck it says TARGET. We need to tell a driver something like “sorry, this delivery is not for us”. In program language it is called conditional statment and the syntax would be similar to this:
if (truckName == “IKEA”) { recieveFurniture() }
else { say (“sorry, this delivery is not for us”)}
There are 4 tables and 3 chairs on the track and all of them for us. Driver gives you a list of the furniture and it looks like this:
listOfFurniture = [table1, table2, table3, table4, chair1, chair2, chair3]

Note: computers start numeration from zero, not from one. So numeration in our array would be like this:

Numeration starts from zero, not from one!!!
Now, when we have an array, we can refer to each piece of our furniture by name of the array and the number.
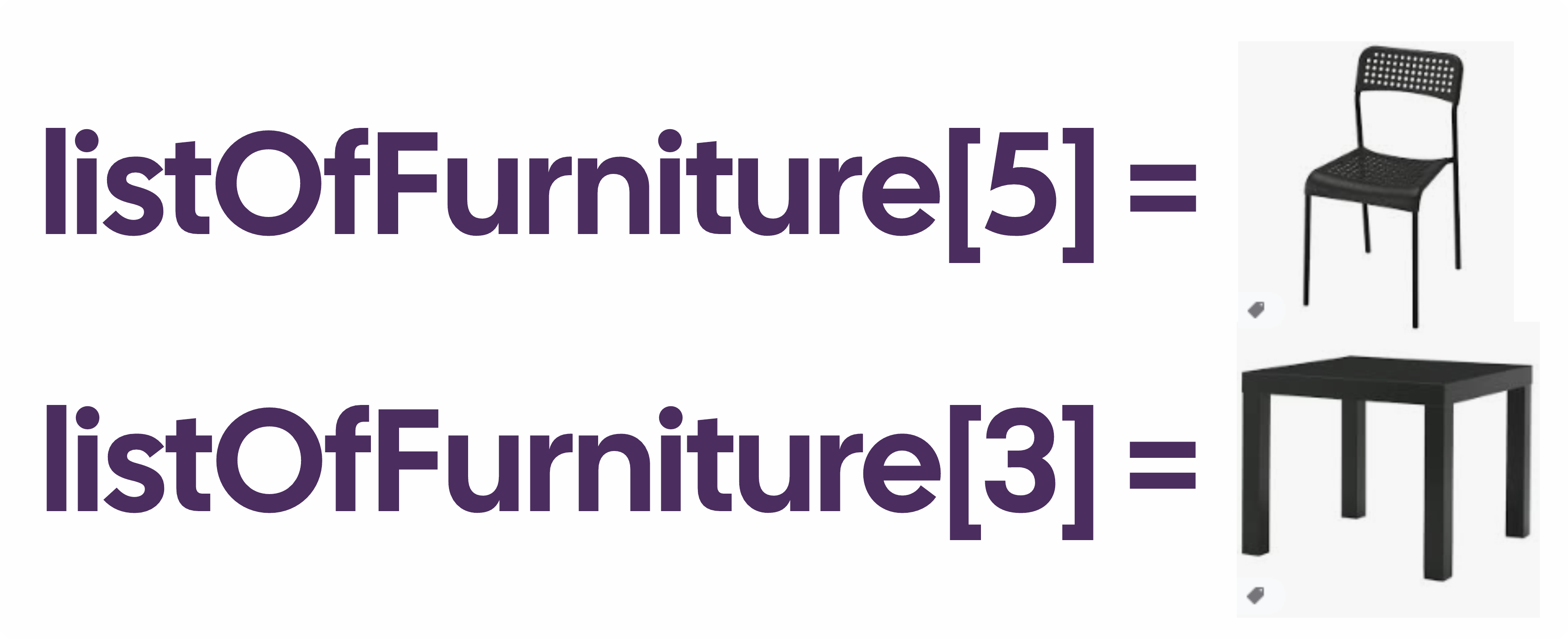
It’s much easier than referring to them by description. Compare “listOfFurniture, number 3” and “black table, the one which is smaller and has chunky legs”
Objects
Along with the list of furniture, the truck driver gives us a bunch of paper, each piece of paper contains detailed information about one piece of the furniture. We will have a look at the description of a random piece of furniture from the list, a black table with chunky legs, for example. The description has a certain format, there are keys (type, name, article number, color, price…) and their values (table for type, LACK for name, 003.529.88 for article number, black for color, $12.90 for price…) and this let us look at the table as an Object.
Each pair is called property. Besides properties, objects may have methods. The syntax for calling methods would be like this:
object.method(parameters) // for methods with parameters
object.method() // if method takes no parameters.
Look at methods like at actions which we can perform on the object. If we go back to our table and imagine what we could do with it we get a list of methods.
pieceOfFurniture.rotate(angle) // will rotate our table on an angle which we pass as a parameter
pieceOfFurniture.paint(color) // will paint the table to chosen color
pieceOfFurniture.turnUpsideDown() // will rotate our table upsidedown. As you can see this method takes no parameters
We access data in our array simply by using the name of the array and the index of the element. Objects have a more complicated structure. To get access to the value of the particular key we would need the name of the object and the name of the key. We also can get a list of all key-value couples or find the name of the key if we know the object name and value of the key. For example, if we want to find all black pieces of furniture in our array we would need to check which objects have a key with name "color" and value "black". We also can take a piece of furniture and check its type, by accessing a value of the key with name "type". Check here to find out more about accessing data in objects.
Control flow and functions
After we had a look at the documentation for our furniture it is time to unload the truck and put each piece of furniture (POF) in its place (all those furniture for the lounge on the second floor). For each table and chair we would need to perform the same set of operations:
Retrieve POF from the truck
Bring POF to the building
Press a lift button
Enter the lift
Press button with number two
Exit the lift
Bring POF to the lounge
Place POF in its place
As we are going to perform the same set of instructions for each piece it is useful to describe it once and then refer to it every time we have a new piece of furniture. This is a function and the syntax would be similar to:
function deliverPOF() {
Retrieve POF from the truck
Bring POF to the building
Press a lift button
Enter the lift
Press button with number two
Exit the lift
Bring POF to the lounge
Place POF in its place
}
If we think we may need the same set of operations, but a different room on the different floor, for another furniture delivery, it is make sense to design our function with parameters:
function deliverPOF(floorNumber,roomName) {
Retrieve POF from the truck
Bring POF to the building
Press a lift button
Enter the lift
Press button with number [floorNumber]
Exit the lift
Bring POF to the [roomName]
Place POF in its place
}
Now when we have a function for each POF we can go through our furniture piece by piece. As we have our furniture in an array, we can use method forEach, which perform function on each element of the array:
forEach (deliverPOF(2,lounge))
2,lounge - are our parameters for this particular set of furniture.
We also could use while loop.
while (numberOfFurnitureInTheTruck > 0) {
Take piece of furniture
Execute deliverPOF(2,lounge) on it
}
The actual syntax of the while loop you can check here. The code above is a simplification designed to give you an understanding of the conception.
The goal of this post is to give you an understanding of the fundamental ideas of JavaScript. With an understanding of those fundamentals, you can easily search up the syntax of the particular elements and create your own script. Happy coding <3